Day 3
I spent all night yesterday trying to fix a bug in my isometric sorting but couldn't figure it out. And then had to think of it all day today. When I finally got home I added lots of print() statements throughout my code and eventually figured it out.Floating point numbers don't work as you would expect at all. Just because x = a + b doesn't mean that x >= a + b, apparently. After using x + 0.1 >= a + b it suddenly started working...
Unfortunately I hit another problem. My algorithm creates a mask for each block it draws and then draws all other blocks in front of it with the mask active. So for a map with 100 blocks I might end up drawing several thousand blocks... which is too much for Python and/or my GPU. So next is figuring out a way to optimize this, probably by trying to pre-sort the blocks and cache the overlapping ones...
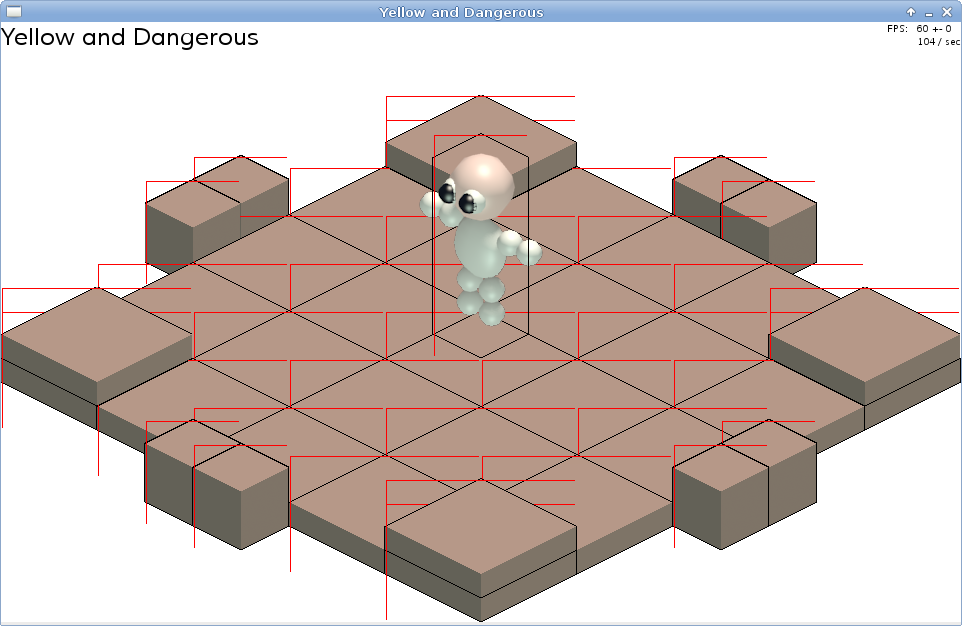
(log in to comment)
Comments
Try this in a Python shell, it's essentially what my bug boiled down to:
>>> s = 96 / 2 ** 0.5
>>> S = -4.75
>>> x0 = S * s
>>> x1 = (S + 1) * s
>>> x0 + s >= x1
False
I "fixed" it by now using:
>>> x0 + s + 0.000000000001 >= x1
True
Not sure why you're making it so complicated. When you have an isometric grid, you can just iterate over the grid in back-to-front order and it should work out.
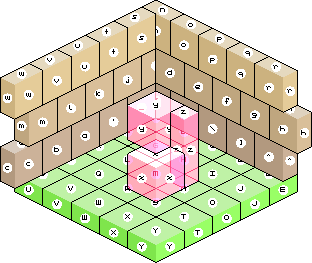
I think z should be split into two halves, each one 1 tile high.
If all blocks are rectangular and have the same height, you could just paint each plane (from floor to ceiling, x before y, b before m, etc.) in ordered manner (from back to front, y before higher half of z, I before O, etc.) and have no problems.
This is just a guess I've never done 3D :)
If all blocks are rectangular and have the same height, you could just paint each plane (from floor to ceiling, x before y, b before m, etc.) in ordered manner (from back to front, y before higher half of z, I before O, etc.) and have no problems.
This is just a guess I've never done 3D :)
I "fixed" it by now using:
>>> x0 + s + 0.000000000001 >= x1
You've just encountered a well known phenomena in floating-point math - the order of operations taken on a floating point number can influence the last few bits of the number enough to throw off any kind of an equality comparison. Normally, that additional factor you're using would be called an "epsilon".
There's an excellent (if rather long) series on floating-point math here:
http://www.cygnus-software.com/papers/comparingfloats/comparingfloats.htm
>>> x0 + s + 0.000000000001 >= x1
You've just encountered a well known phenomena in floating-point math - the order of operations taken on a floating point number can influence the last few bits of the number enough to throw off any kind of an equality comparison. Normally, that additional factor you're using would be called an "epsilon".
There's an excellent (if rather long) series on floating-point math here:
http://www.cygnus-software.com/papers/comparingfloats/comparingfloats.htm
Er... that's not how floating point numbers work. If the first condition is true, the second one will be true. Your issue must have been somewhere else.